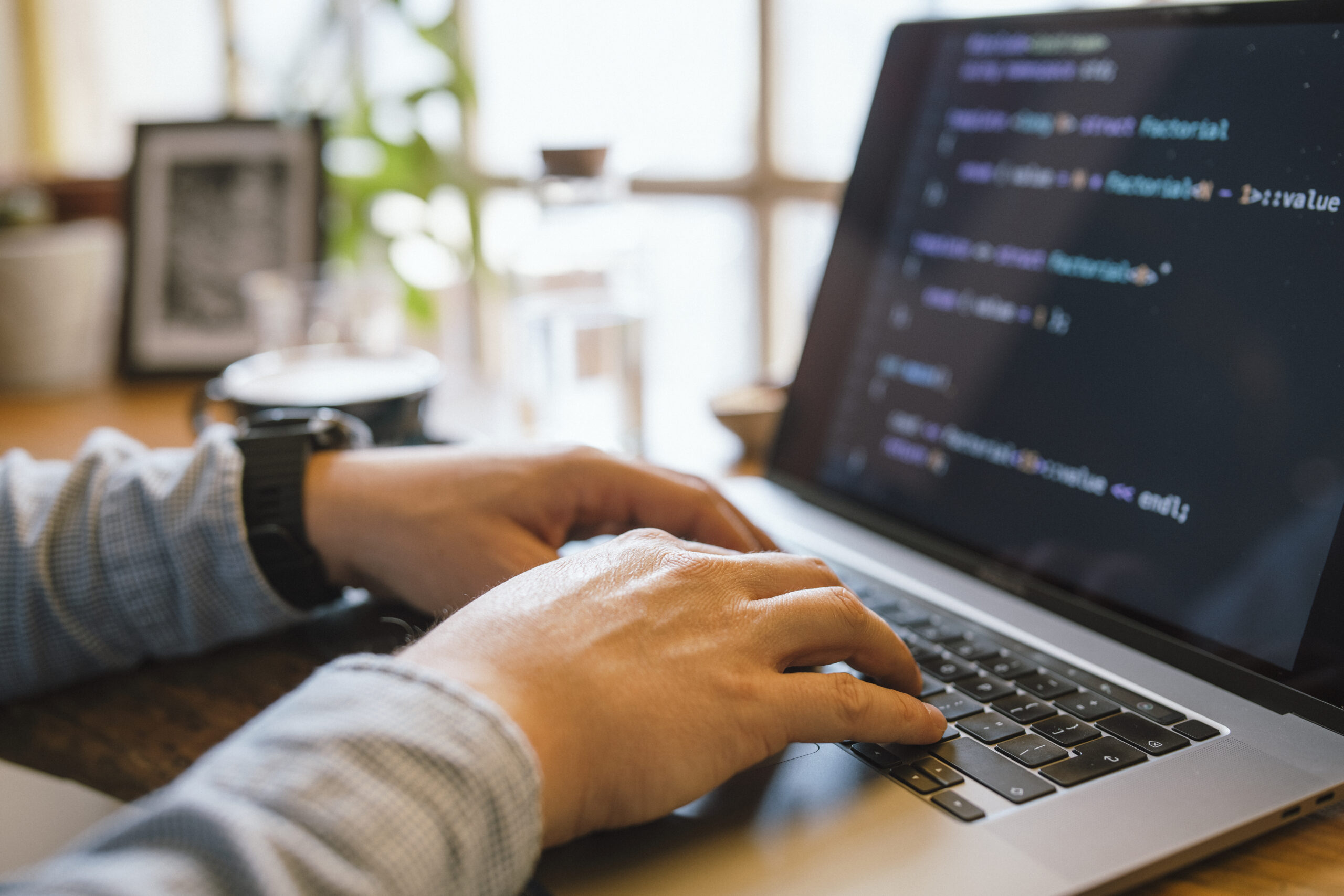
Debugging is Probably the most crucial — nonetheless often ignored — capabilities in a very developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why things go Incorrect, and Understanding to Feel methodically to resolve troubles proficiently. No matter whether you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can help save hrs of stress and substantially transform your productiveness. Allow me to share many approaches to aid developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest ways builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person Component of growth, realizing how you can connect with it properly in the course of execution is equally essential. Modern progress environments arrive equipped with effective debugging capabilities — but quite a few developers only scratch the surface of what these instruments can do.
Choose, by way of example, an Integrated Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These equipment permit you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code within the fly. When used accurately, they Allow you to notice specifically how your code behaves during execution, that's a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They help you inspect the DOM, keep track of community requests, view actual-time general performance metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can flip discouraging UI issues into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate more than managing procedures and memory administration. Studying these equipment could possibly have a steeper learning curve but pays off when debugging efficiency troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Regulate units like Git to know code historical past, uncover the precise minute bugs had been launched, and isolate problematic alterations.
In the long run, mastering your applications means going beyond default options and shortcuts — it’s about establishing an personal familiarity with your progress natural environment to make sure that when issues crop up, you’re not lost in the dark. The greater you know your tools, the greater time you could expend resolving the particular dilemma as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often missed — techniques in productive debugging is reproducing the challenge. In advance of leaping in to the code or making guesses, builders will need to make a constant environment or state of affairs the place the bug reliably appears. Without reproducibility, correcting a bug gets a sport of chance, normally resulting in wasted time and fragile code variations.
Step one in reproducing a problem is accumulating as much context as possible. Check with queries like: What steps resulted in the issue? Which natural environment was it in — progress, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the easier it gets to isolate the exact conditions underneath which the bug occurs.
When you finally’ve collected plenty of info, endeavor to recreate the trouble in your neighborhood surroundings. This may imply inputting the exact same information, simulating very similar user interactions, or mimicking technique states. If The difficulty appears intermittently, look at writing automated assessments that replicate the edge situations or point out transitions concerned. These assessments don't just assist expose the situation but also avoid regressions Down the road.
Occasionally, The problem may very well be surroundings-precise — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Applying resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It demands persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment a lot more properly, examination likely fixes safely and securely, and converse far more Plainly using your crew or consumers. It turns an abstract grievance right into a concrete problem — and that’s exactly where developers prosper.
Examine and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when one thing goes Improper. Instead of seeing them as frustrating interruptions, builders need to find out to treat mistake messages as immediate communications from your method. They often show you what exactly occurred, where it transpired, and often even why it occurred — if you know the way to interpret them.
Start out by reading through the message thoroughly and in full. Quite a few developers, specially when underneath time strain, look at the primary line and right away start generating assumptions. But deeper from the error stack or logs may perhaps lie the real root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — go through and understand them 1st.
Break the error down into pieces. Could it be a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a specific file and line variety? What module or function induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also useful to be aware of the terminology from the programming language or framework you’re working with. Mistake messages in languages like Python, JavaScript, or Java usually observe predictable patterns, and Finding out to acknowledge these can substantially increase your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake happened. Verify relevant log entries, enter values, and up to date changes inside the codebase.
Don’t forget about compiler or linter warnings possibly. These often precede greater troubles and supply hints about opportunity bugs.
Ultimately, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint troubles speedier, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an software behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging technique starts with understanding what to log and at what level. Common logging concentrations involve DEBUG, Details, Alert, Mistake, and FATAL. Use DEBUG for comprehensive diagnostic information during enhancement, Details for normal functions (like productive begin-ups), Alert for probable troubles that don’t break the applying, Mistake for true issues, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. Too much logging can obscure vital messages and slow down your system. Deal with essential functions, state variations, input/output values, and critical final decision points in the code.
Format your log messages clearly and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. Using a very well-thought-out logging strategy, you could reduce the time it requires to identify challenges, acquire further visibility into your purposes, and improve the All round maintainability and dependability within your code.
Believe Like a Detective
Debugging is not simply a complex endeavor—it's a type of investigation. To properly establish and fix bugs, developers should strategy the method just like a detective resolving a secret. This mentality helps break down complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Get started by gathering proof. Think about the symptoms of the issue: error messages, incorrect output, or overall performance problems. Much like a detective surveys a crime scene, acquire as much pertinent details as you'll be able to with no jumping to conclusions. Use logs, check instances, and user reports to piece alongside one another a transparent picture of what’s going on.
Future, variety hypotheses. Check with by yourself: What may be leading to this conduct? Have any modifications recently been built to your codebase? Has this situation transpired prior to under identical situation? The aim would be to slender down options and discover possible culprits.
Then, exam your theories systematically. Endeavor to recreate the trouble inside a managed surroundings. In the event you suspect a specific perform or component, isolate it and validate if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the final results lead you nearer to the truth.
Pay back near attention to smaller particulars. Bugs normally conceal in the minimum expected spots—like a missing semicolon, an off-by-one error, or a race issue. Be thorough and client, resisting the urge to patch the issue with no fully knowledge it. Short-term fixes may well hide the true trouble, only for it to resurface later on.
Last of all, preserve notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging system can conserve time for long run problems and support others fully grasp your reasoning.
By thinking like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and develop into more practical at uncovering hidden problems in intricate units.
Write Exams
Composing assessments is among the simplest ways to enhance your debugging expertise and Over-all development efficiency. Exams not just support capture bugs early but will also function a security Web that provides you self confidence when building variations to your codebase. A nicely-tested application is easier to debug because it permits you to pinpoint just the place and when a difficulty happens.
Begin with unit tests, which concentrate on person functions or modules. These small, isolated checks can immediately expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a test fails, you immediately know where to glimpse, appreciably cutting down time invested debugging. Device assessments are Specially valuable for catching regression bugs—concerns that reappear following Beforehand staying mounted.
Subsequent, combine integration assessments and stop-to-end checks into your workflow. These support make certain that numerous aspects of your application function alongside one another easily. They’re especially practical for catching bugs that come about in sophisticated systems with many elements or products and services interacting. If a thing breaks, your tests can inform you which Portion of the pipeline unsuccessful and below what disorders.
Composing tests also forces you to Imagine critically about your code. To check a function thoroughly, you will need to understand its inputs, predicted outputs, and edge conditions. This amount of being familiar with By natural means potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. After the take a look at fails regularly, it is possible to deal with fixing the bug and look at your exam pass when The problem is solved. This solution ensures that the identical bug doesn’t return Sooner or later.
In short, creating exams turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—helping you catch much more bugs, more rapidly plus much more reliably.
Take Breaks
When debugging check here a tricky problem, it’s straightforward to be immersed in the situation—gazing your monitor for several hours, trying Option just after Alternative. But one of the most underrated debugging resources is simply stepping away. Taking breaks assists you reset your thoughts, decrease disappointment, and often see the issue from a new perspective.
If you're much too near the code for much too extensive, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code that you just wrote just hrs previously. Within this state, your Mind will become a lot less successful at dilemma-solving. A short wander, a coffee break, or even switching to another undertaking for ten–15 minutes can refresh your target. Numerous builders report acquiring the basis of an issue when they've taken the perfect time to disconnect, permitting their subconscious operate within the background.
Breaks also enable avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You may instantly observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you prior to.
For those who’re caught, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a five–10 moment break. Use that time to maneuver close to, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular under restricted deadlines, but it in fact causes more quickly and more practical debugging In the end.
Briefly, having breaks will not be an indication of weakness—it’s a wise system. It gives your brain Place to breathe, increases your viewpoint, and can help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Each individual bug you encounter is much more than simply A short lived setback—It is really an opportunity to increase for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing useful in case you make the effort to replicate and review what went wrong.
Begin by asking by yourself a number of critical thoughts as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit testing, code reviews, or logging? The responses usually reveal blind spots in your workflow or understanding and assist you to Develop stronger coding habits moving ahead.
Documenting bugs can even be an outstanding routine. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Eventually, you’ll begin to see designs—recurring concerns or common issues—you could proactively prevent.
In crew environments, sharing Everything you've learned from the bug using your peers is usually In particular strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast understanding-sharing session, helping Some others stay away from the same situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a few of the finest developers are certainly not the ones who publish perfect code, but individuals who continuously find out from their issues.
Ultimately, Each individual bug you resolve provides a new layer in your talent established. So up coming time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, more capable developer thanks to it.
Conclusion
Bettering your debugging techniques takes time, follow, and endurance — though the payoff is huge. It helps make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.